Installation
Install Tailwind CSS with Phoenix
Setting up Tailwind CSS in a Phoenix project.
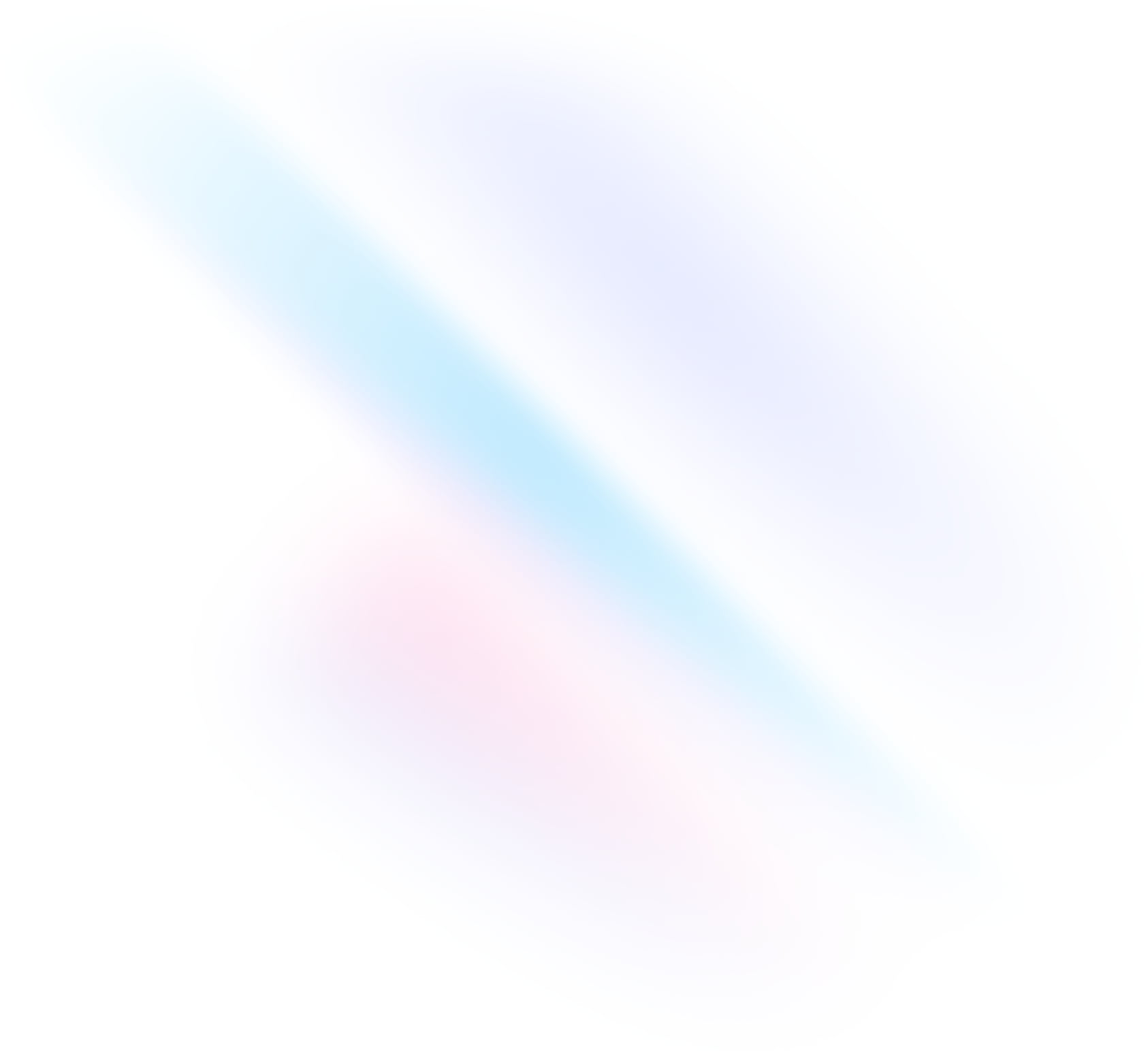
Create your project
Start by creating a new Phoenix project if you don't have one set up already. You can follow their installation guide to get up and running.
Terminalmix phx.new myprojectcd myproject
Install the Tailwind plugin
Add the Tailwind plugin to your dependencies and run
mix deps.get
to install it.mix.exsdefp deps do [ {:tailwind, "~> 0.1", runtime: Mix.env() == :dev} ] end
Configure the Tailwind plugin
In your
config.exs
file you can set which version of Tailwind CSS you want to use, the path to your Tailwind config, and customize your asset paths.config.exsconfig :tailwind, version: "3.4.17", default: [ args: ~w( --config=tailwind.config.js --input=css/app.css --output=../priv/static/assets/app.css ), cd: Path.expand("../assets", __DIR__) ]
Update your deployment script
Configure your
assets.deploy
alias to build your CSS on deployment.mix.exsdefp aliases do [ setup: ["deps.get", "ecto.setup"], "ecto.setup": ["ecto.create", "ecto.migrate", "run priv/repo/seeds.exs"], "ecto.reset": ["ecto.drop", "ecto.setup"], test: ["ecto.create --quiet", "ecto.migrate --quiet", "test"], "assets.deploy": ["tailwind default --minify", "esbuild default --minify", "phx.digest"] ] end
Enable watcher in development
Add Tailwind to your list of watchers in your
./config/dev.exs
file.dev.exswatchers: [ # Start the esbuild watcher by calling Esbuild.install_and_run(:default, args) esbuild: {Esbuild, :install_and_run, [:default, ~w(--sourcemap=inline --watch)]}, tailwind: {Tailwind, :install_and_run, [:default, ~w(--watch)]} ]
Install Tailwind CSS
Run the install command to download the standalone Tailwind CLI and generate a
tailwind.config.js
file in the./assets
directory.Terminalmix tailwind.install
Configure your template paths
Add the paths to all of your template files in your
./assets/tailwind.config.js
file.tailwind.config.js/** @type {import('tailwindcss').Config} */ module.exports = { content: [ './js/**/*.js', '../lib/*_web.ex', '../lib/*_web/**/*.*ex', ], theme: { extend: {}, }, plugins: [], }
Add the Tailwind directives to your CSS
Add the
@tailwind
directives for each of Tailwind’s layers to./assets/css/app.css
app.css@tailwind base; @tailwind components; @tailwind utilities;
Remove the default CSS import
Remove the CSS import from
./assets/js/app.js
, as Tailwind is now handling this for you.app.js// Remove this line if you add your own CSS build pipeline (e.g postcss). import "../css/app.css"
Start your build process
Run your build process with
mix phx.server
.Terminalmix phx.server
Start using Tailwind in your project
Start using Tailwind’s utility classes to style your content.
index.html.heex<h1 class="text-3xl font-bold underline"> Hello world! </h1>